An iOS Bundle is just a folder which contains resources. Roughly speaking, it allows you to store any resource you might need: images, nibs, or localized string files.
Why would you create an application bundle?. What’s that good for?.
Suppose you have a framework of your own. And… suppose that your framework has several UIViewController which require custom images. OR, suppose that you need to load different images dynamically, within your framework’s code.
You can’t include those resources inside an iOS framework. So… that’s where the bundles come in!.
Check out the Facebook SDK. Yup. It contains a bundle with few image resources.
[Step #1] : Adding a Bundle Target
Let’s add a new target to our project, which will be the one responsible of generating the bundle.
First of all, we need to click over the project name in the navigator (1), and afterwards, over the ‘add target’ button at the bottom (2):
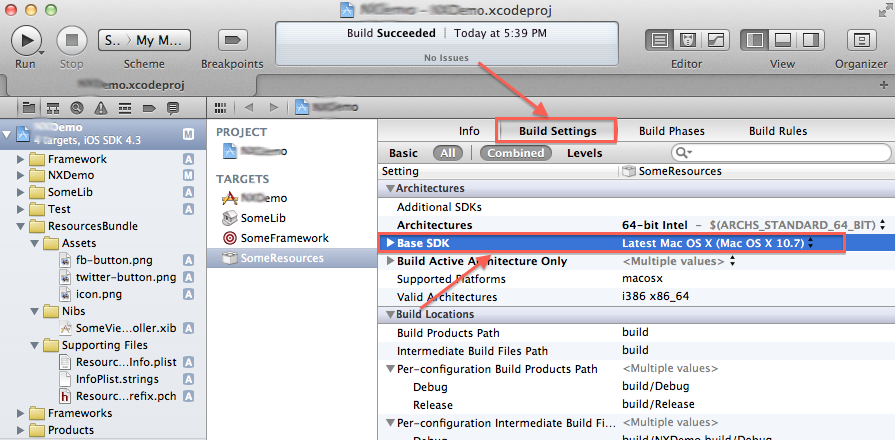
You can find the ‘Bundle’ target in the following path: ‘Mac OSX >> Framework & Library >> Bundle’:
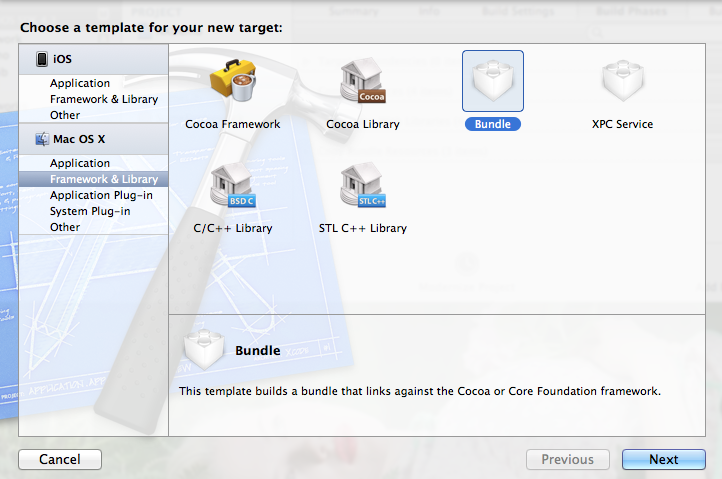
Did you notice that we had to use the ‘Bundle’ target-template located inside MacOSX section?. Aaaand… did you notice that there is no Bundle target-template inside the iOS section?.
OSX and iOS bundles have a slightly different structure. We can use any of them both. But since we’re working on iOS, let’s switch to its own Bundle structure.
We need to change the ‘base sdk’ Build Setting for our new SomeResources target, as follows:
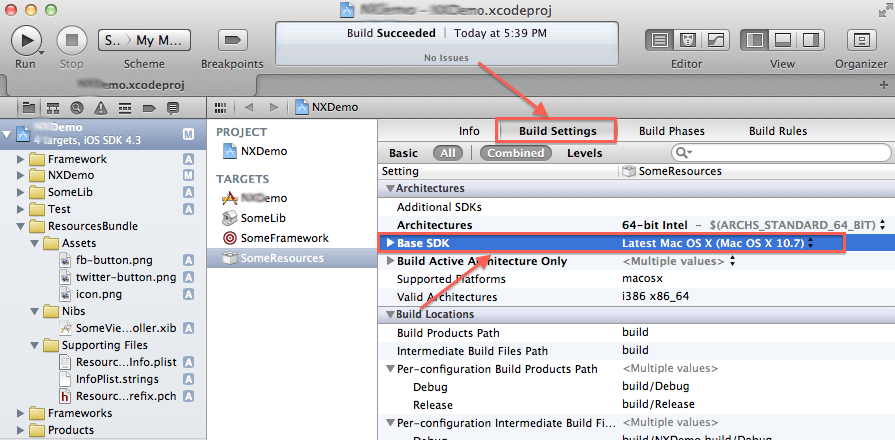
Let’s update the BaseSDK. Instead of Latest Mac OSX, please, click over that combo and select ‘Latest iOS’.
Allright!. It’s time to edit the Build Phases section of our Bundle. We’ve named our new target as ‘SomeResources’. We just need to add all of our resources into the ‘Copy Bundle Resources’ section, as shown below:
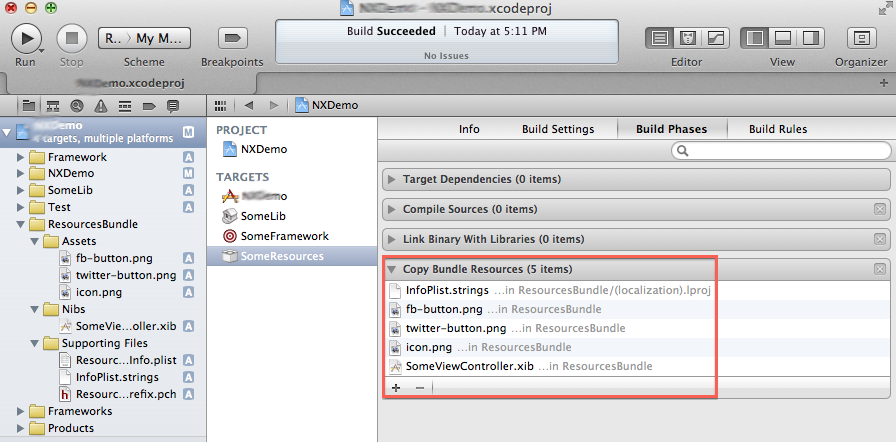
Note that, for this particular example, we’re exporting three PNG images, a NIB file, and an InfoPlist.strings file. We’ll see, later on, how to load these files.
In order to generate the bundle, we just need to select the ‘SomeResources’ schema:
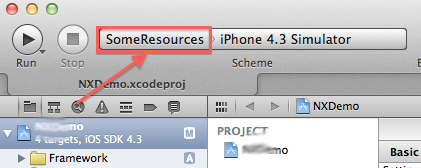
Once we have the ‘SomeResources’ schema selected, we must hit “build” in order to generate the bundle file.
Your build-output folder should look as follows, assuming you’re in debug mode. ‘SomeResources.bundle’ is ready to be distributed:
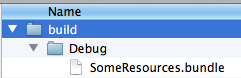
[Step #2] : Including SomeResources.bundle into a Sample project
How do we manage to import this new bundle into a new project?. Simply drag & drop the ‘SomeResources.bundle’ file into your target app’s Resources folder, as shown below. That’s all you need to do.
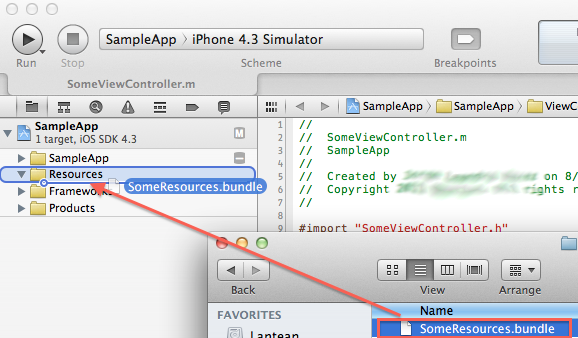
Up next… we’ll learn how to:
I hope you found this mini guide useful… somehow!.
Have fun!.
Like this:
Like Loading...