It’s been a while since the last iPhone jailbreak was made public. But all evil comes to an end, sooner rather than later. A couple days ago, a hacking group named evad3rs, published a brand new Jailbreak tool for iOS 6.x.
It’s claimed to be able to jailbreak any iOS device: iPod / iPad / iPhone / iPad Mini. I’ve tested it myself on two devices: iPhone 4s and iPhone 5. The results were impressive. They managed to combine at least 3 different exploits to make things work.
The process is quite seamless. You simply download the app, run it, and follow the instructions onscreen. The binary is available for OSX, Windows and even Linux.
You can download it here. What to do once the device is jailbroken?. You can perform an incredible amount of customizations, that are not available in the pristine version of iOS.
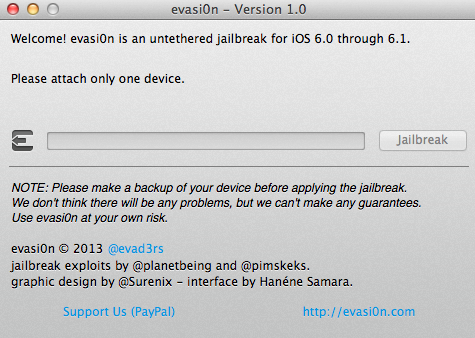
If, on the contrary, you’re still running an old version of iOS, we suggest you check out this site. They’ve got a nice repository of information with the previous jailbreaks that were made available… who doesn’t remember BlackRa1n, LimeRa1n… JailbreakMe or GreenPoison?.
Those were golden times, but Apple has spent a lot on hardening iOS security (no wonder why they’re now providing devices to the US Government, while RIM is directed right ahead to chapter eleven).
By the way.. we do not encourage the usage of hacked / cracked software. If you like an app, please, support the devs!.
Like this:
Like Loading...